Exposing Addin Functions to Javascript
Addins can expose Android functions to the browser environment, which can be called directly from JavaScript. In order to do this, first implement the IJavascriptInterface
interface:
private IJavascriptInterface mJsInterface = new IJavascriptInterface() {
@Override
public String getInterfaceName()
return "TestAddin"
}
@JavascriptInterface
public String getRandomString() {
return UUID.randomUUID().toString(); //JavaScript: var r = TestAddin.getRandomString();
}
};
The @JavascriptInterface
annotation allows your method to by exposed to the browser. Your exposed functions will be accessible in a class that is named by your getInterfaceName()
method.
Next, add the interface to an array and return that array from the IKioWareAddin.getJavascriptInterfaces()
method:
@Override
public IJavascriptInterface[] getJavascriptInterfaces() {
return new IJavascriptInterface[] { this.mJsInterface };
}
We recommend only returning simple types, such as void, int, decimal, boolean, and String. For complex types, we recommend using a JSON library to serialize the object in the addin, and then calling JSON.parse() in the browser to deserialize the object. Here's the sample function we'll use:
@JavascriptInterface
public String getRandomString() {
return TestAddin.getRandomString();
}
Your new function can now be called from the browser using the following JavaScript code:
<a href="#" onclick="javascript:alert(TestAddin.getRandomString());">Try It</a>
If your function performs functionality that would be dangerous for any domain/page on the internet to be able to execute, you can require KioWare to make sure the page is listed in the Securty Access List, with higher trust requirements. To require a specific level of trust, update your Scripting Access Control List to include your domains and pages as appropriate. Replace "Your Domain" with the domain or domains you wish to allow, omitting the quotations:
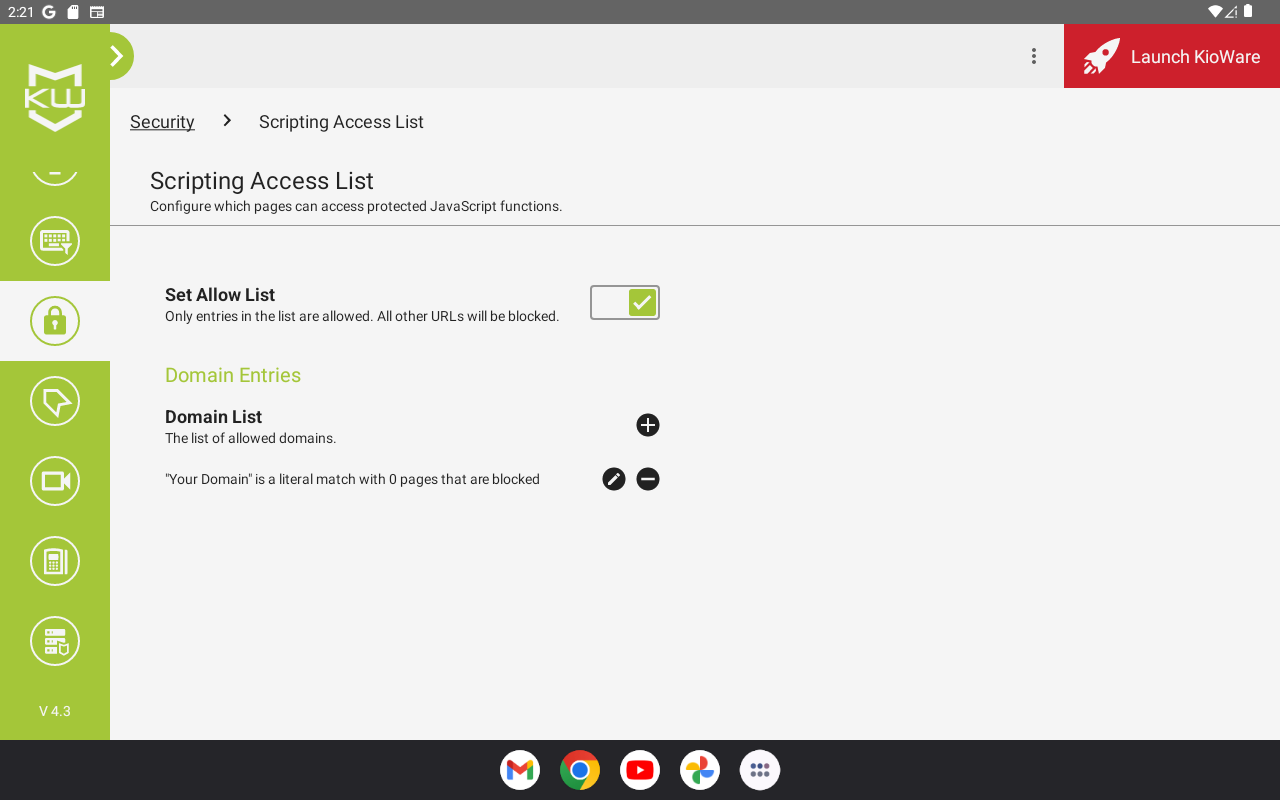